Reference :http://www.codeproject.com/Tips/597253/Using-the-Grid-MVC-in-ASP-NET-MVC
FirsT step: create a new ASP.NET MVC 4 application, I recommended to use the basic template.
Next, lets create a new class Client, this class will be the model:
Before create the view, will need to add a Grid.MVC package using Nuget:

Also add Boostrap:

When the Grid.MVC package is installed, you can found some new views in the Views/Shared folder:
In the scripts folder, tree new JavaScript files will be found:

And in the content folder now you see the css file for the Grid:
Next step now is to create a new View for the action Index of the controller Client, like this:
Index
The key parts on the code are:
FirsT step: create a new ASP.NET MVC 4 application, I recommended to use the basic template.
Next, lets create a new class Client, this class will be the model:
public class Client
{
public int Id { get; set; }
public string Name { get; set; }
public string Email { get; set; }
}
Now that you have the model done, lets create the Client controller.
This controller have only on action, and the data source will be a List,
but here you can connect to a database, the action returns the data:public class ClientController : Controller
{
private readonly List clients = new List()
{
new Client { Id = 1, Name = "Julio Avellaneda", Email = "julito_gtu@hotmail.com" },
new Client { Id = 2, Name = "Juan Torres", Email = "jtorres@hotmail.com" },
new Client { Id = 3, Name = "Oscar Camacho", Email = "oscar@hotmail.com" },
new Client { Id = 4, Name = "Gina Urrego", Email = "ginna@hotmail.com" },
new Client { Id = 5, Name = "Nathalia Ramirez", Email = "natha@hotmail.com" },
new Client { Id = 6, Name = "Raul Rodriguez", Email = "rodriguez.raul@hotmail.com" },
new Client { Id = 7, Name = "Johana Espitia", Email = "johana_espitia@hotmail.com" }
};
public ActionResult Index()
{
return View(clients);
}
}
Before create the view, will need to add a Grid.MVC package using Nuget:

Also add Boostrap:

When the Grid.MVC package is installed, you can found some new views in the Views/Shared folder:

In the scripts folder, tree new JavaScript files will be found:

And in the content folder now you see the css file for the Grid:
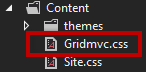
Next step now is to create a new View for the action Index of the controller Client, like this:
@model IEnumerable
@using GridMvc.Html
@{
Layout = null;
}
"
viewport" content="width=device-width" />
"@Url.Content("~/Content/Gridmvc.css")" rel="stylesheet" />
"@Url.Content("~/Content/bootstrap.min.css")" rel="stylesheet" />
Grid.MVC
"
width:500px;">
@Html.Grid(Model).Columns(columns =>
{
columns.Add(c => c.Id).Titled("Client ID");
columns.Add(c => c.Name).Titled("Name").Filterable(true);
columns.Add(c => c.Email).Titled("Email");
}).WithPaging(3).Sortable(true)
The key parts on the code are:
- Add: @using GridMvc.Html
- Add the references to the css files
- Add the references to the JavaScript files
- Titled: The column title
- Filterable: Define if the column has the feature to be filterable
- Sortable: Define if the column has the feature to be sortable
- WithPaging: Define the number of rows to show for page

Comments